Components
<ChangeEmailCard />
The <ChangeEmailCard />
component provides a simple and secure UI that allows users to change their account email address, including verification emails sent to the updated address, if email verification is enabled.
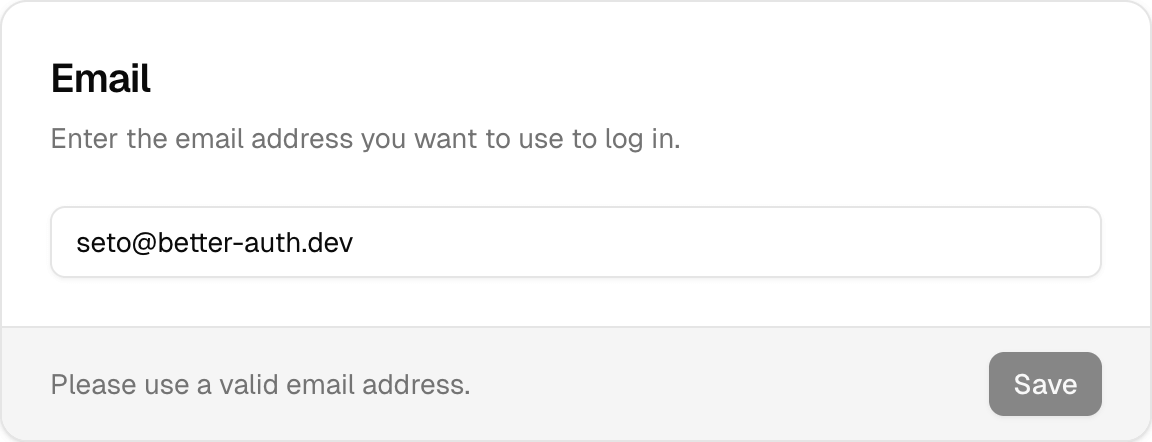
Usage
Here's how to implement <ChangeEmailCard />
on your custom settings page:
The component automatically integrates with your AuthUIProvider
context and handles email verification workflows seamlessly.
Reference
These are the available props for <ChangeEmailCard />
:
Prop | Type | Default |
---|---|---|
className? | string | - |
classNames? | SettingsCardClassNames | - |
isPending? | boolean | - |
localization? | Partial<{ account: string; accounts: string; accountsDescription: string; accountsInstructions: string; addAccount: string; addPasskey: string; alreadyHaveAnAccount: string; avatar: string; avatarDescription: string; ... 131 more ...; passwordTooShort: string; }> | - |
Styling
You can customize the styles using the provided classNames
prop:
Localization
You can pass custom text via the localization
prop: